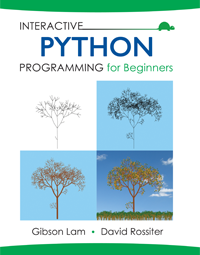
Interactive Python Programming
for Beginners
for Beginners
ISBN: | 978-9-814-74296-2 |
---|---|
Publisher: | McGraw Hill Education |
Year: | 2016 |
Pages: | 248 |
Code Examples
Please click on each chapter on the left to show the examples.
-
Chapter 1 Introduction to Python
- Code Listing 1.1 - first_program.py
-
Chapter 2 Basic Turtle Graphics Programming
- Code Listing 2.1 - moving_forward.py
- Code Listing 2.2 - equilateral_triangle.py
- Code Listing 2.3 - simple_square.py
- Code Listing 2.4 - disconnected_circles.py
- Code Listing 2.5 - archery_target.py
- Code Listing 2.6 - solid_triangle.py
- Code Listing 2.7 - solid_star.py
-
Chapter 3 Comments, Variables and Text
- Code Listing 3.1 - writing_comments.py
- Code Listing 3.2 - using_variables.py
- Code Listing 3.3 - printing_separate_lines.py
- Code Listing 3.4 - printing_same_line.py
- Code Listing 3.5 - printing_comma_separated.py
- Code Listing 3.6 - using_tabs.py
- Code Listing 3.7 - using_newlines.py
- Code Listing 3.8 - using_input_with_error.py
- Code Listing 3.9 - using_input_without_error.py
-
Chapter 4 Variable Types and Operators
- Code Listing 4.1 - letter_b.py
-
Chapter 5 Making Decisions
- Code Listing 5.1 - colour_ball.py
- Code Listing 5.2 - colour_ball_using_elif.py
-
Chapter 6 Looping
- Code Listing 6.1 - drawing_square_using_while_loop.py
- Code Listing 6.2 - regular_polygons.py
- Code Listing 6.3 - infinite_loop.py
- Code Listing 6.4 - dotted_triangle.py
- Code Listing 6.5 - drawing_square_using_for_loop.py
- Code Listing 6.6 - using_underscore.py
- Code Listing 6.7 - spiral_pattern.py
- Code Listing 6.8 - flower_of_hexagons.py
- Code Listing 6.9 - printing_five_numbers.py
- Code Listing 6.10 - using_continue.py
- Code Listing 6.11 - using_break.py
-
Chapter 7 Finding Repeating Patterns
- Code Listing 7.1 - odd_or_even_number.py
- Code Listing 7.2 - finding_leap_years.py
- Code Listing 7.3 - alternate_grey_columns.py
- Code Listing 7.4 - chess_board.py
-
Chapter 8 Lists, Tuples and Strings
- Code Listing 8.1 - rainbow.py
- Code Listing 8.2 - using_len.py
- Code Listing 8.3 and Code Listing 8.4 - telephone_directory.py
-
Chapter 9 Functions
- Code Listing 9.1 - drawing_square_using_function.py
- Code Listing 9.2 - window_pattern.py
- Code Listing 9.3 - window_pattern_with_colour.py
- Code Listing 9.4 - searching_telephone_directory.py
- Code Listing 9.5 - different_variable_names.py
- Code Listing 9.6 - magic_trick_not_working.py
- Code Listing 9.7 - magic_trick_with_error.py
- Code Listing 9.8 - magic_trick_working.py
-
Chapter 11 More on Turtle
- Code Listing 11.1 - drawing_squares_with_different_speed.py
- Code Listing 11.2 - turning_animation_off.py
- Code Listing 11.3 - drawing_squares_without_animation.py
- Code Listing 11.4 - drawing_squares_using_four_colours.py
- Code Listing 11.5 - earth_and_moon.py
-
Chapter 12 Turtle Window Event Handling
- Code Listing 12.1 - turning_turtle_using_click_event.py
- Code Listing 12.2 - dragging_turtle_using_drag_event.py
- Code Listing 12.3 - dragging_turtle_using_goto.py
- Code Listing 12.4 - dragging_turtle_without_animation.py
- Code Listing 12.5 - using_multiple_turtles.py
- Code Listing 12.6 - moving_turtle_without_drawing.py
- Code Listing 12.7 - drawing_program.py
- Code Listing 12.8 - controlling_turtle_using_key_events.py
- Code Listing 12.9 - simple_timer.py
- Code Listing 12.10 - two_timers.py
- Code Listing 12.11 - stopwatch_program.py
-
Chapter 13 File Handling
- Code Listing 13.1 - controlling_turtle_with_keys_and_memory.py
- Code Listing 13.2 - controlling_turtle_and_saving_data.py
- Code Listing 13.3 - controlling_turtle_and_loading_data.py
-
Chapter 14 Programming with Objects
- Code Listing 14.1 and Code Listing 14.2 - drawing_red_circle_using_circle_class.py
- Code Listing 14.1 and Code Listing 14.3 - drawing_random_circles_using_circle_class.py
-
Chapter 15 Recursion
- Code Listing 15.1 - drawing_recursive_tree.py