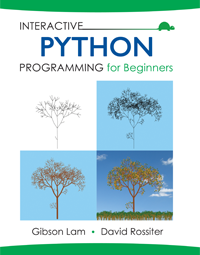
Interactive Python Programming
for Beginners
for Beginners
ISBN: | 978-9-814-74296-2 |
---|---|
Publisher: | McGraw Hill Education |
Year: | 2016 |
Pages: | 248 |
Exercise Answers
Please click on each chapter on the left to show the answers of the exercises at the back of each chapter.
-
Chapter 2 Basic Turtle Graphics Programming
Exercise 2.1 - A turtle and a turtle window. Exercise 2.2 - turtle.home()
,turtle.goto(0,0)
andturtle.reset()
.Exercise 2.3 - turtle.setheading(90)
andturtle.right(270)
.Exercise 2.4 - Two parallel vertical lines are drawn in the turtle window. Exercise 2.5 - archery_target_using_circles.py Exercise 2.6 - archery_target_using_dots.py Exercise 2.7 - A hollow circle with a black border. Exercise 2.8 - (The program in the question contains mistakes. Please refer to the errata for the corrections.)
A black dot in the middle of the window.Exercise 2.9 - tic_tac_toe.py Exercise 2.10 - drawing_dots.py -
Chapter 3 Comments, Variables and Text
Exercise 3.1 - hollow_star.py Exercise 3.2 - 10
Exercise 3.3 - c Exercise 3.4 - equilateral_triangle.py Exercise 3.5 - c Exercise 3.6 - It can be done by joining the text using \n
in a singleprint
command, i.e.:
printing_multiple_lines.pyExercise 3.7 - printing_party_stuff.py Exercise 3.8 - No, they are not the same because the single line of code would produce a space before the question mark. Exercise 3.9 - whats_your_name.py -
Chapter 4 Variable Types and Operators
Exercise 4.1 - - i. an integer
- ii. a string
- iii. a float
- iv. a string
Exercise 4.2 - You are 21.0 years old.
Exercise 4.3 - An error is shown because the int
command cannot convert a floating point number to an integer.Exercise 4.4 - If a float is stored in a variable float_number
, the following code rounds the float to the nearest integer:
int(float_number + 0.5)
Exercise 4.5 - If a float is stored in a variable float_number
, the following code extracts the fractional part from the number:
float_number - int(float_number)
Exercise 4.6 - My lucky number is 777.
Exercise 4.7 - 1
-
Chapter 5 Making Decisions
Exercise 5.1 - First, a colon has to be added at the end of the if
statement and second, the comparison oftime
and9
should use==
instead of=
, i.e. the line containing theif
statement should look like this:
if time == 9:
Exercise 5.2 - - i.
False
- ii.
True
Exercise 5.3 - Yes, it is correctly indented. Exercise 5.4 - No, it is not. Exercise 5.5 - Python is the best!
Exercise 5.6 - programming_languages.py Exercise 5.7 - Here are two of the possible conditions:
x >= 1 and x <= 999
x > 0 and x < 1000
Exercise 5.8 - Here is a possible extension:
x >= 1 and x <= 999 and x % 5 != 0
Exercise 5.9 - bmi_calculation.py - i.
-
Chapter 6 Looping
Exercise 6.1 - solid_star_with_while_loop.py Exercise 6.2 - There are five black dots lining up horizontally. Exercise 6.3 - solid_star_with_for_loop.py Exercise 6.4 - - i. 0
- ii. 0, 10, 20, 30, 40, 50, 60, 70, 80 and 90
- iii. 100, 90, 80, 70, 60, 50, 40, 30, 20 and 10
- iv. Nothing
Exercise 6.5 - hollow_star.py Exercise 6.6 - finding_prime_number.py Exercise 6.7 - The capital letter A Exercise 6.8 - (The program in the question has a wrong range. Please refer to the errata for the corrections.)
- i.
>
- ii.
<=
- iii.
!=
Exercise 6.9 - square_pattern.py Exercise 6.10 - c -
Chapter 7 Finding Repeating Patterns
Exercise 7.1 - number_places.py Exercise 7.2 - The condition checks whether the difference between the current hour and the last hour is divisible by 4 like this:
if (hour - last_hour) % 4 == 0:
Exercise 7.3 - The condition in the first line of code inside the for loop is:
if (row + column) % 3 == 0:
-
Chapter 8 Lists, Tuples and Strings
Exercise 8.1 - printing_book_title.py Exercise 8.2 - ['apple', 'orange', 'banana']
Exercise 8.3 - A triangle is on the left and a square on the right. Exercise 8.4 - - i. An error is produced
- ii.
(1, 2, 1, 2, 1, 2)
- iii.
[5, 4, 3, 2, 1]
Exercise 8.5 - LOL
Exercise 8.6 - rainbow_text.py Exercise 8.7 - - i.
aaa
- ii. An empty line
- iii. An empty line
Exercise 8.8 - hyappppayh
-
Chapter 9 Functions
Exercise 9.1 - regular_polygon.py Exercise 9.2 - finding_max.py Exercise 9.3 - finding_max_from_variables.py Exercise 9.4 - quadratic_equation.py Exercise 9.5 - The adjusted score is still 49 because the function only changes the local variable score
without changing the content of the global variablescore
. -
Chapter 10 Dictionaries
Exercise 10.1 - students = {"10042": "Mary", "10067": "John", "12029": "Andy", "11047": "Molly", "12334": "Jane"}
Exercise 10.2 - searching_students.py Exercise 10.3 - color_fruits.py Exercise 10.4 - 10 -
Chapter 11 More on Turtle
Exercise 11.1 - (The program in the question contains a mistake. Please refer to the errata for the correction.)
The major difference is thatturtle.tracer(True)
turns on the turtle animation whereasturtle.update()
does not.Exercise 11.2 - displaying_python.py Exercise 11.3 - The turtle moves to a new position which is 50 pixels to the right and 100 pixels above of the current position. Exercise 11.4 - drawing_oval.py Exercise 11.5 - sun_earth_moon.py Exercise 11.6 - Five circles of different colours, in the order of red, yellow, green, blue and purple, are drawn across the turtle window. -
Chapter 12 Turtle Window Event Handling
Exercise 12.1 - Yes. Exercise 12.2 - improved_drawing_program.py Exercise 12.3 - traffic_lights.py Exercise 12.4 - rotating_moon.py Exercise 12.5 - rotating_moon_with_control.py Exercise 12.6 - The following line of code should be added inside the if statement:
turtle.ontimer(countdown, 1000)
Exercise 12.7 - The following line of code should be added inside the for loop:
turtle.ontimer(countdown, (number + 1) * 1000)
-
Chapter 13 File Handling
Exercise 13.1 - "r"
means opening a file for reading.
"w"
means opening a file for writing and the content of the file will be overwritten.
"a"
means opening a file for writing and the new content will be added at the end of the existing content.Exercise 13.2 - Nothing is shown because each line of text in the file is stored in the list together with the newline character at the end. That means "Mary"
is stored as"Mary\n"
inside the listnames
.Exercise 13.3 - 123456789
Exercise 13.4 - drawing_program_with_file.py -
Chapter 14 Programming with Objects
Exercise 14.1 - Here are some example attributes for the class: - -
studentid
- -
firstname
- -
lastname
- -
gender
- -
department
- -
enroll(course)
- -
attend(class)
- -
join(club)
Exercise 14.2 - dog_class.py Exercise 14.3 - extended_circle_class.py Exercise 14.4 - extended_circle_class_2.py Exercise 14.5 - square_class.py Exercise 14.6 - multiple_random_shapes.py - -
-
Chapter 15 Recursion
Exercise 15.1 - recursive_formula.py Exercise 15.2 - Exercise 15.3 - 8 Exercise 15.4 - nohtyP
Exercise 15.5 - 3